Downloaded the following packages via SourceForge:
- Sweet Home 3D - most popular Java program on SourceForge. Very elaborate interior decorating application that allows you to create a house from scratch. One of the first features one comes across is the ability to place a window on a solid wall, which allows one to see thru the window into the interior room.
- Risk - the popular board game coded in Java. Impressive. One can even play in network mode.
- sQuirrel SQL Client - a java database connect program. This was the only program that was downloaded as a .jar on my Windows 7 machine. Installation produced an error. Maybe if I was being paid to make it work I would've spent more time on it, but I'm not, so I moved on to the next application.
- The Art of Illusion - a fully-featured 3D Java program. I installed this on my mac and immediately thought, "wow this looks a lot like a monochrome scratchy version of maya."
I decided to review Risk. It was a toss up between that and Sweet Home 3D, but I figured 1) why do the most popular one and 2) Risk seemed like the more masculine choice. And Risk seemed to have better source documentation. And I like board games.
Here's my evaluation based on the three primitive directives:
- The system successfully accomplishes a useful task
- An external user can successfully install and use the system
- An external developer can successfully understand and enhance the system
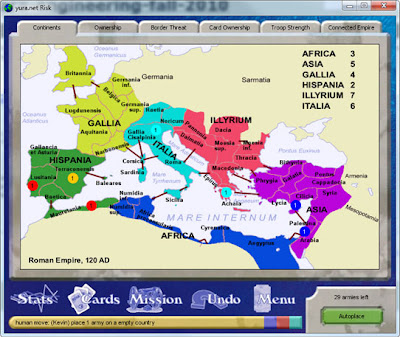
PRIMITIVE DIRECTIVE 1:
- Smooth and intuitive gameplay that allows a plethora of options. Amazingly, it even offers network play, which I did not have an opportunity to test. I think the best compliment here is that one hardly notices the GUI at all. Very well done and no noticeable bugs to slow down or frustrate the user.
- Some nice options, like computer AI-levels, as well as different maps to play on. E.g., Ancient Rome, Axis and Allies, Risk 2210, etc.
PRIMITIVE DIRECTIVE 2:
- Downloading/Installing - I installed this game on both OSX (Snow Leopard) and Windows 7. The download script was smart enough to download a .jar file for the mac and an .exe file for Windows. Installation on the mac seemed to go a little smoother. On Windows 7, the installation gave me a warning that the application may not have installed properly, but I was able to run it without any problems.
- Usability/User Manual/Documentation - Excellent. Not the prettiest of user manuals, but basically contains everything the user needs to know about the game, from gameplay, to the intuitive GUI, in addition to guides to the different GUIs (Flash vs. Java Swing GUI). The game itself is very intuitive, and if the user is familiar with Risk at all the manual is practically a luxury.
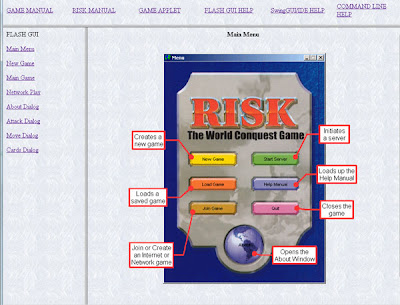
PRIMITIVE DIRECTIVE 3:
- Source Files - The installation asks the user if the java source files should be installed. Upon installation, the source files are included in a .zip file and upon extraction, one finds that the code has been nicely modularized into packages, folders, and classes. This was a three-person collaboration (according to the game credits).
- Importing Files into Eclipse - This caused some hassle. The project did not import nicely into Eclipse and after 2 hours of trying to resolve the 2500+ errors that Eclipse found upon import, I have to assume that the developers used another IDE - or I may be missing a crucial setting in the import. All of the errors seem to be from the naming scheme used by Eclipse upon importing the 136 files that the application is composed of. None of the import statements and package statements seem to have imported properly, thus causing type definition errors.
- Conclusion: Overall, however, the organization of the code seems very well organized. The game engine has it's own package, as does the computer AI, and the main method is easy to locate (it's found in the GUI interface files). All class names also make sense, and it's intuitive what most of the classes do (E.g., Card.java, Continent.java, Mission.java, Statistic.java, etc.). The code seems to be easy to modify or change due to its modularity and organization.